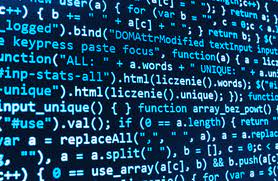
在当今互联网时代,Web安全已经成为开发者必须重视的核心议题。本文将从实践角度出发,深入介绍Web安全威胁与防护措施。
常见的Web安全威胁
1. XSS (跨站脚本攻击)
XSS攻击通过在网页中注入恶意脚本来获取用户信息或执行未经授权的操作。
1.1 存储型XSS
攻击原理: 攻击者将恶意脚本存储在目标服务器上(如评论、用户资料等),其他用户访问页面时触发执行
危害: 可以窃取用户cookie、篡改页面内容、记录用户键盘输入等
防护措施:
对用户输入进行严格过滤和转义
1
2
3
4
5
6
7
8
9// 基本HTML转义函数示例
function escapeHtml(unsafe) {
return unsafe
.replace(/&/g, "&")
.replace(/</g, "<")
.replace(/>/g, ">")
.replace(/"/g, """)
.replace(/'/g, "'");
}使用成熟的XSS过滤库,如DOMPurify
设置响应头
Content-Security-Policy
对输出进行编码,使用模板引擎的安全特性
1.2 反射型XSS
- 攻击原理: 攻击者构造特殊URL,诱导用户点击,服务器将URL中的恶意脚本返回给用户执行
- 危害: 可以执行任意JavaScript代码,获取用户敏感信息
- 防护措施:
- 对URL参数进行严格校验
- 使用
httpOnly
Cookie - 实现输入验证白名单
- 设置
X-XSS-Protection
响应头
1.3 DOM型XSS
攻击原理: 纯客户端的XSS,通过修改DOM树结构来实现攻击
危害: 可以操作DOM、窃取用户信息
防护措施:
避免使用
innerHTML
,优先使用textContent
使用安全的DOM API
1
2
3
4
5// 不安全的写法
element.innerHTML = userInput;
// 安全的写法
element.textContent = userInput;对DOM操作相关的数据进行严格校验
使用 React/Vue 等框架的安全特性
2. 注入攻击
2.1 SQL注入
- 攻击原理: 通过构造特殊的SQL语句,破坏原有SQL语句结构
- 攻击示例:
1
2
3
4
5
6
7
8
9-- 原始SQL
SELECT * FROM users WHERE username = '${username}' AND password = '${password}'
-- 注入攻击
username: admin' --
password: anything
-- 最终执行的SQL
SELECT * FROM users WHERE username = 'admin' -- ' AND password = 'anything'
防护措施:
使用参数化查询
1
2
3
4
5
6
7
8
9
10
11// Node.js MySQL示例
const mysql = require('mysql2');
// 不安全的写法
const query = `SELECT * FROM users WHERE id = ${userId}`;
// 安全的写法
const query = 'SELECT * FROM users WHERE id = ?';
connection.execute(query, [userId], (err, results) => {
// 处理结果
});ORM框架
最小权限原则
输入验证和转义
3. CSRF (跨站请求伪造)
3.1 GET类型CSRF
- 攻击原理: 诱导用户点击特制URL,利用用户登录态执行操作
- 攻击示例:
1
2<!-- 攻击页面 -->
<img src="http://bank.example/transfer?amount=1000&to=attacker" />
防护措施:
使用CSRF Token
1
2
3
4
5
6
7// Express.js示例
const csrf = require('csurf');
app.use(csrf({ cookie: true }));
app.get('/form', (req, res) => {
res.render('form', { csrfToken: req.csrfToken() });
});验证请求来源
1
2
3
4
5
6
7
8
9// 检查Referer头
app.use((req, res, next) => {
const referer = req.headers.referer;
if (referer && new URL(referer).origin === 'https://yoursite.com') {
next();
} else {
res.status(403).send('Invalid referer');
}
});SameSite Cookie属性
使用POST替代GET请求
安全检测与监控
1. 自动化安全扫描
使用OWASP ZAP进行漏洞扫描
1
2
3
4# 使用Docker运行ZAP扫描
docker run -v $(pwd):/zap/wrk/:rw -t owasp/zap2docker-stable zap-baseline.py \
-t https://your-target-website.com \
-r scan-report.html代码安全审计工具
1
2
3
4
5
6
7
8
9
10
11
12
13
14// 使用ESLint的安全规则
{
"extends": [
"plugin:security/recommended"
],
"plugins": [
"security"
],
"rules": {
"security/detect-eval-with-expression": "error",
"security/detect-non-literal-regexp": "error",
"security/detect-unsafe-regex": "error"
}
}
2. 日志监控
记录异常登录行为
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22// Winston日志配置示例
const winston = require('winston');
const securityLogger = winston.createLogger({
format: winston.format.json(),
transports: [
new winston.transports.File({
filename: 'security.log',
level: 'warn'
})
]
});
// 记录登录失败
function logFailedLogin(username, ip) {
securityLogger.warn('Failed login attempt', {
username,
ip,
timestamp: new Date().toISOString(),
event: 'LOGIN_FAILED'
});
}监控敏感操作
1
2
3
4
5
6
7
8
9
10
11
12
13
14// Express中间件示例
const sensitiveOps = new Set(['/admin', '/api/users', '/api/delete']);
app.use((req, res, next) => {
if (sensitiveOps.has(req.path)) {
securityLogger.info('Sensitive operation', {
path: req.path,
method: req.method,
user: req.user?.id,
ip: req.ip
});
}
next();
});告警系统集成
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21// 钉钉告警示例
async function sendSecurityAlert(message) {
const webhook = 'https://oapi.dingtalk.com/robot/send?access_token=xxx';
await axios.post(webhook, {
msgtype: 'text',
text: {
content: `🚨安全告警:${message}`
}
});
}
// 设置告警阈值
const LOGIN_FAIL_THRESHOLD = 5;
const timeWindow = new Map(); // IP -> 失败次数
function checkLoginAttempts(ip) {
const fails = timeWindow.get(ip) || 0;
if (fails >= LOGIN_FAIL_THRESHOLD) {
sendSecurityAlert(`IP ${ip} 在5分钟内登录失败超过${LOGIN_FAIL_THRESHOLD}次`);
}
}
3. 应急响应
制定应急预案
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23// 安全事件等级定义
const SecurityLevel = {
LOW: {
name: '低危',
responseTime: '24小时',
notifyChannels: ['email']
},
MEDIUM: {
name: '中危',
responseTime: '12小时',
notifyChannels: ['email', 'phone']
},
HIGH: {
name: '高危',
responseTime: '2小时',
notifyChannels: ['email', 'phone', 'sms']
},
CRITICAL: {
name: '严重',
responseTime: '30分钟',
notifyChannels: ['email', 'phone', 'sms', 'callCenter']
}
};安全事件处理流程
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36// 事件处理状态机
const EventStatus = {
DETECTED: 'detected',
CONFIRMED: 'confirmed',
ANALYZING: 'analyzing',
FIXING: 'fixing',
VERIFIED: 'verified',
CLOSED: 'closed'
};
class SecurityIncident {
constructor(level, description) {
this.id = uuid();
this.level = level;
this.description = description;
this.status = EventStatus.DETECTED;
this.timeline = [{
status: EventStatus.DETECTED,
timestamp: new Date(),
operator: 'system'
}];
}
async escalate() {
// 升级处理
}
async notify() {
// 通知相关人员
}
async archive() {
// 归档处理
this.generateReport();
}
}
总结
Web安全是一个需要持续投入的系统工程,需要在以下方面持续努力:
- 代码层面
- 遵循安全编码规范
1
2
3
4
5
6
7
8
9
10
11
12
13
14// 密码加密最佳实践
const bcrypt = require('bcrypt');
async function hashPassword(password) {
const salt = await bcrypt.genSalt(10);
return bcrypt.hash(password, salt);
}
// 安全的随机数生成
const crypto = require('crypto');
function generateSecureToken(length = 32) {
return crypto.randomBytes(length).toString('hex');
}
- 遵循安全编码规范
- 使用成熟的安全库
1
2
3
4
5
6
7
8
9
10
11
12
13
14// Helmet.js 安全头配置
const helmet = require('helmet');
app.use(helmet({
contentSecurityPolicy: {
directives: {
defaultSrc: ["'self'"],
scriptSrc: ["'self'", "'unsafe-inline'"],
styleSrc: ["'self'", "'unsafe-inline'"],
imgSrc: ["'self'", "data:", "https:"],
},
},
referrerPolicy: { policy: 'same-origin' }
}));
- 架构层面
- 最小权限原则
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22// 基于角色的访问控制(RBAC)
const roles = {
ADMIN: ['read', 'write', 'delete'],
EDITOR: ['read', 'write'],
VIEWER: ['read']
};
function checkPermission(user, action) {
const userRole = user.role;
return roles[userRole]?.includes(action) || false;
}
// 权限中间件
function requirePermission(action) {
return (req, res, next) => {
if (checkPermission(req.user, action)) {
next();
} else {
res.status(403).json({ error: '权限不足' });
}
};
}
- 最小权限原则
- 纵深防御策略
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21// 1. 请求频率限制
const rateLimit = require('express-rate-limit');
const apiLimiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15分钟
max: 100, // 限制100次请求
message: '请求过于频繁,请稍后再试'
});
app.use('/api/', apiLimiter);
// 2. IP黑名单
const blacklist = new Set();
app.use((req, res, next) => {
const clientIP = req.ip;
if (blacklist.has(clientIP)) {
return res.status(403).send('Access Denied');
}
next();
});
- 运维层面
- 自动化安全检查
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27# GitHub Actions安全扫描配置
name: Security Scan
on:
push:
branches: [ main ]
pull_request:
branches: [ main ]
jobs:
security:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Run OWASP ZAP Scan
uses: zaproxy/action-baseline@v0.4.0
with:
target: 'https://your-staging-app.com'
- name: Run npm audit
run: npm audit
- name: Run Snyk Security Scan
uses: snyk/actions/node@master
env:
SNYK_TOKEN: ${{ secrets.SNYK_TOKEN }}
- 自动化安全检查
- 监控告警配置
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19// 监控系统集成
const monitor = {
metrics: {
loginFailures: new Map(), // IP -> 失败次数
apiErrors: new Map(), // 接口 -> 错误次数
slowRequests: new Map() // 路径 -> 慢请求次数
},
async check() {
for (const [metric, thresholds] of Object.entries(this.thresholds)) {
const value = await this.getValue(metric);
if (value > thresholds.critical) {
this.notify('critical', `${metric} 超过临界值: ${value}`);
} else if (value > thresholds.warning) {
this.notify('warning', `${metric} 超过警戒值: ${value}`);
}
}
}
};
这些最佳实践示例都是基于实际项目经验总结的,可以直接应用到生产环境中。每个示例都包含了详细的注释和配置说明,方便开发者理解和使用。